Inheritance can be defined as the process where one class acquires the properties of another class.
Properties – Methods and Fields…
Aim: Reusability of code
So that rest of the common properties and functionalities can be extended from the another class.
In the Inheritance we can consider any two class as
1. Child Class
2. Parent Class
1. Child Class- The class that extends the features of another class
It is also known as sub class or derived class.
2. Parent Class – The class whose properties and functionalities are used(inherited) by another class.
It is also known as parent class, super class or base class.
Advantage of using Inheritance:
Code which is present in base class need not be rewritten in the child class.
Syntax of Inheritance:
class ABC extends JKL{ } // Here, ABC is a child class and JKL is a parent class
Example Program For Inheritance:
class Car {
String name = “Car”;
String company = “Maruti”;
void demo(){
System.out.println(“Car is New”);
}
}
public class BMW extends Car{
String mainCompany = “BMW”;
public static void main(String args[]){
BMW obj = new BMW();
System.out.println(obj.company);
System.out.println(obj.name);
System.out.println(obj.mainCompany);
obj.demo();
}
}
Output: Maruti, Car, BMW
Types of Inheritance in Java:
1. Single level Inheritance: It refers to a child and parent class relationship where a class extends the another class.
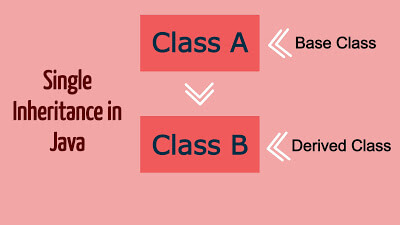
Example Program For Single Inheritance:
class one {
public void abc()
{
System.out.println(“Java”);
}
}
class two extends one {
public void xyz()
{
System.out.println(“Android”);
}
}
public class Main
{
public static void main(String[] args)
{
two g = new two();
g.abc();
g.xyz();
}
}
Output: Java, Android
2.Multilevel Inheritance: It refers to a child and parent class relationship where a class extends the child class.
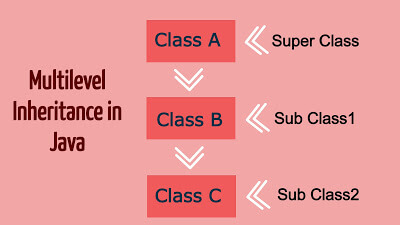
Example Program For Multilevel Inheritance:
class one {
public void abc()
{
System.out.println(“Learn”);
}
}
class two extends one {
public void xyz()
{
System.out.println(“Java”);
}
}
class three extends two {
public void jkl()
{
System.out.println(“Android”);
}
}
public class Main1
{
public static void main(String[] args)
{
three g = new three();
g.abc();
g.xyz();
g.jkl();
}
}
Output: Learn, Java, Android
3. Hierarchical Inheritance: It refers to a child and parent class relationship where more than one classes extends the same class.
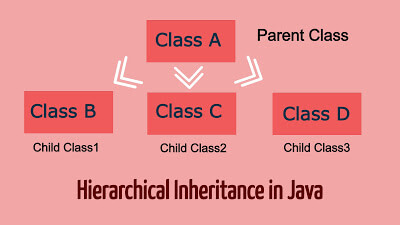
Example Program For Hierarchical Inheritance:
class one {
public void abc()
{
System.out.println(“Learn”);
}
}
class two extends one {
public void xyz()
{
System.out.println(“Java”);
}
}
class three extends one {
public void jkl()
{
}
}
public class Main2
{
public static void main(String[] args)
{
three g = new three();
g.abc();
two t = new two();
t.xyz();
g.jkl();
}
}
Output: Learn, Java
4. Multiple Inheritance: It refers to the concept of one class extending more than one classes, which means a child class has two parent classes as you can see on the screen. The thing is Java doesn’t support multiple inheritance. In java, we can achieve multiple inheritance only through Interfaces.
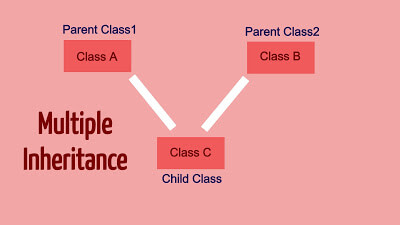
5. Hybrid Inheritance: Hybrid Inheritance is a Combination of more than one types of inheritance in a single program. Since java doesn’t support multiple inheritance with classes, the hybrid inheritance is also not possible with classes.