1. Right Triangle Pattern In Java
public class RightTrianglePattern{
public static void main(String[] args){
int i, j;
int rows = 5;
for(i = 1; i<= rows; i++){ // for number of rows
for(j = 1; j<=i; ++j){
System.out.print("*"); // this will print the stars
}
System.out.println(); // finally from here it will go to the next line
}
}
}
Output:
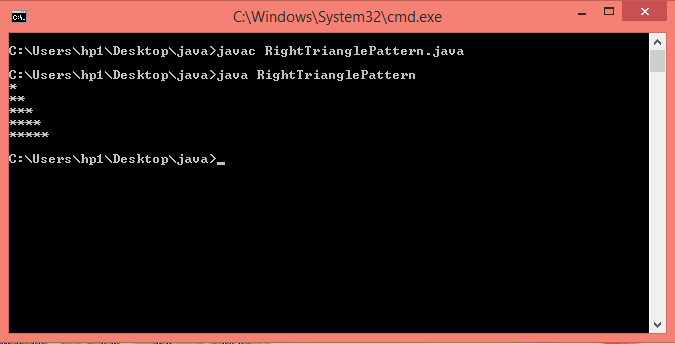
2. Pyramid Pattern In Java
public class PyramidPattern{
public static void main(String[] args){
int n = 5; // for number of rows
printPyramid(n); // create a local method
}
public static void printPyramid(int n){
for(int i = 0; i<n; i++){ // to handle the number of spaces
for(int j = n-i; j>1; j--){
System.out.print(" "); // this will print the spaces
}
for(int j = 0; j<=i; j++){
System.out.print("* "); // this will print the stars
}
System.out.println(); // finally from here it will come to the next line
}
}
}
Output:
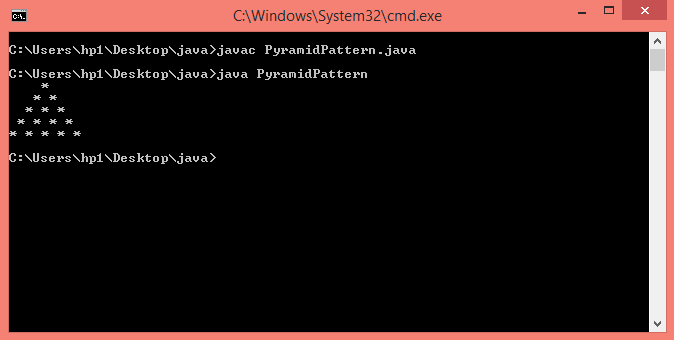
3. Left Triangle Pattern In Java
public class LeftTrianglePattern{
public static void main(String[] args){
for(int i =1; i<=5; i++){ // for number of rows
for(int j =1; j<= 5-i+1; j++){
System.out.print(" "); // to handle number of spaces
}
for(int j =1; j<=i; j++){
System.out.print("*"); // this will print the stars
}
System.out.println(); // finally from here, it will go the next line
}
}
}
Output:
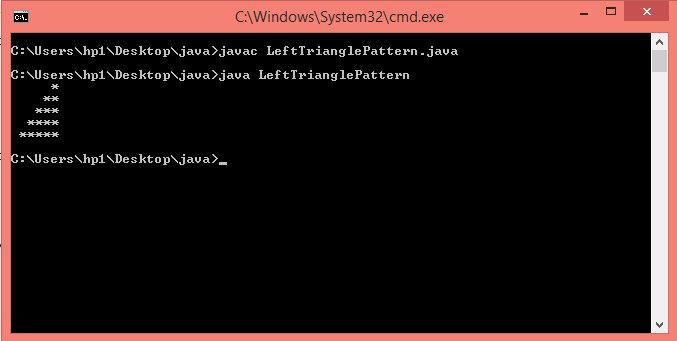
Watch the video for step by step tutorial:
MORE CORE JAVA PROGRAMS
- What is Interface In Java and What are its Uses?
- Java Scanner Class(User Input) with Example
- This, Super and Final keywords in Java with Examples
- What is Encapsulation in java | Java Encapsultion | Getters and Setters
- Abstraction | Java Abstraction OOPs | Abstract Class and Method
- What is Polymorphism in Java | Java Polymorphism
- What is Inheritance in Java? Java Inheritance types
- What is Constructors in Java | Java Constructor
- Loops in Java | Java Tutorial | For | While | For-Each | Do-While
- What is hashmap in java | mapping in java | hashmap java